How To Upload Exe As A Web Application
Editor's note: This article was last updated 24 March 2022 to reflect updates to Node.js and the trunk-parser
library.
Multer is a Node.js middleware for treatment multipart/form-data
that makes the otherwise painstaking process of uploading files in Node.js much easier. In this commodity, we'll larn the purpose of Multer in handling files in submitted forms. Nosotros'll too explore Multer by building a mini app with a frontend and backend to test uploading a file. Let's get started!
Table of contents
- Managing user inputs in forms
- Encoding and uploading forms with Multer
- Multer: an overview
- Building an app with Multer support
- Creating our frontend
- Install and configure Multer
- Determination
Managing user inputs in forms
Spider web applications receive all different types of input from users, including text, graphical controls like checkboxes or radio buttons, and files, like images, videos, and other media.
In forms, each of these inputs are submitted to a server that processes the inputs, uses them in some way, perhaps saving them somewhere else, then gives the frontend a success
or failed
response.
When submitting forms that contain text inputs, the server, Node.js in our case, has less piece of work to do. Using Limited, yous tin can easily take hold of all the inputs entered in the req.body
object. However, submitting forms with files is a fleck more complex because they require more processing, which is where Multer comes in.
Encoding and uploading forms with Multer
All forms include an enctype
attribute, which specifies how data should be encoded by the browser earlier sending it to the server. The default value is application/x-www-form-urlencoded
, which supports alphanumeric information. The other encoding blazon is multipart/form-data
, which involves uploading files through forms.
There are 2 ways to upload forms with multipart/form-data
encoding. The get-go is by using the enctype
aspect:
<form activity='/upload_files' enctype='multipart/form-data'> ... </grade>
The code above sends the form-data to the /upload_files
path of your application. The second is past using the FormData
API. The FormData
API allows us to build a multipart/form-information
form with cardinal-value pairs that can exist sent to the server. Here'southward how information technology's used:
const form = new FormData() form.append('name', "Dillion") form.append('paradigm', <a file>)
On sending such forms, information technology becomes the server'south responsibility to correctly parse the form and execute the last operation on the data.
Multer: an overview
Multer is a middleware designed to handle multipart/class-data
in forms. Information technology is similar to the popular Node.js torso-parser
, which is built into Express middleware for course submissions. But, Multer differs in that it supports multipart information, only processing multipart/form-data
forms.
Multer does the work of trunk-parser
by attaching the values of text fields in the req.body
object. Multer also creates a new object for multiple files, eitherreq.file
or req.files
, which holds information about those files. From the file object, you can pick whatever data is required to mail the file to a media management API, like Cloudinary.
Now that we understand the importance of Multer, we'll build a pocket-sized sample app to show how a frontend app tin can send iii different files at once in a form, and how Multer is able to process the files on the backend, making them bachelor for further use.
Building an app with Multer support
We'll start by edifice the frontend using vanilla HTML, CSS, and JavaScript. Of form, you tin hands utilize whatsoever framework to follow forth.
Creating our frontend
Kickoff, create a binder chosen file-upload-example
, and so create another folder chosen frontend
within. In the frontend binder, nosotros'll have three standard files, index.html
, styles.css
, and script.js
:
<!-- index.html --> <body> <div class="container"> <h1>File Upload</h1> <grade id='grade'> <div class="input-grouping"> <label for='name'>Your name</characterization> <input name='name' id='name' placeholder="Enter your name" /> </div> <div class="input-grouping"> <label for='files'>Select files</label> <input id='files' type="file" multiple> </div> <push form="submit-btn" type='submit'>Upload</button> </course> </div> <script src='./script.js'></script> </body>
Observe that we've created a label and input for Your Name
as well every bit Select Files
. We likewise added an Upload
button.
Side by side, we'll add together the CSS for styling:
/* mode.css */ body { background-color: rgb(6, 26, 27); } * { box-sizing: border-box; } .container { max-width: 500px; margin: 60px auto; } .container h1 { text-align: center; color: white; } course { background-colour: white; padding: 30px; } form .input-group { margin-lesser: 15px; } form label { display: block; margin-lesser: 10px; } course input { padding: 12px 20px; width: 100%; border: 1px solid #ccc; } .submit-btn { width: 100%; edge: none; background: rgb(37, 83, 3); font-size: 18px; color: white; border-radius: 3px; padding: 20px; text-align: heart; }
Below is a screenshot of the webpage and so far:
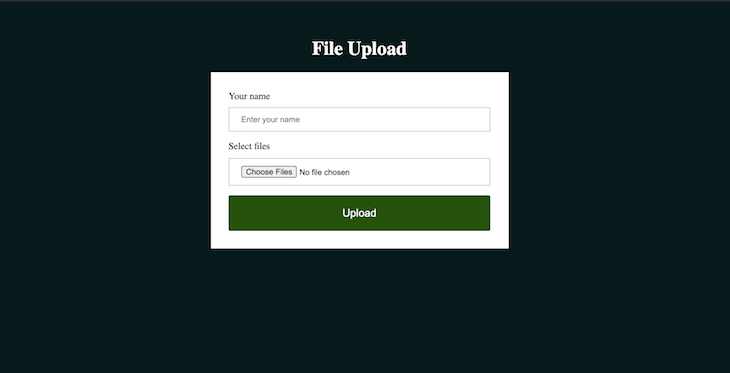
Every bit you can see, the form nosotros created takes two inputs, proper noun
and files
. The multiple
attribute specified in the files
input enables united states of america to select multiple files.
Next, we'll send the form to the server using the lawmaking below:
// script.js const class = document.getElementById("grade"); form.addEventListener("submit", submitForm); function submitForm(eastward) { e.preventDefault(); const proper name = document.getElementById("name"); const files = document.getElementById("files"); const formData = new FormData(); formData.suspend("name", name.value); for(let i =0; i < files.files.length; i++) { formData.append("files", files.files[i]); } fetch("http://localhost:5000/upload_files", { method: 'POST', body: formData, headers: { "Content-Blazon": "multipart/class-data" } }) .then((res) => panel.log(res)) .take hold of((err) => ("Fault occured", err)); }
There are several of import things that must happen when nosotros utilize script.js
. First, we go the grade
element from the DOM and add a submit
event to it. Upon submitting, we use preventDefault
to foreclose the default activity that the browser would take when a form is submitted, which would normally be redirecting to the value of the action
attribute. Next, we go the name
and files
input element from the DOM and createformData.
From here, nosotros'll suspend the value of the name input using a key of name
to the formData
. And then, nosotros dynamically add the multiple files nosotros selected to the formData
using a key of files
.
Note: if nosotros're only concerned with a single file, we can append
files.files[0]
.
Finally, we'll add a POST
asking to http://localhost:5000/upload_files
, which is the API on the backend that nosotros'll build in the next department.
Setting upward the server
For our demo, we'll build our backend using Node.js and Express. Nosotros'll set up a simple API in upload_files
and beginning our server on localhost:5000
. The API will receive a Post
request that contains the inputs from the submitted form.
To employ Node.js for our server, we'll need to ready a bones Node.js projection. In the root directory of the projection in the terminal at file-upload-example
, run the following code:
npm init -y
The command higher up creates a basic package.json
with some information almost your app. Side by side, we'll install the required dependency, which for our purposes is Express:
npm i express
Adjacent, create a server.js
file and add the following lawmaking:
// server.js const express = require("express"); const app = limited(); app.utilise(express.json()); app.use(express.urlencoded({ extended: truthful })); app.postal service("/upload_files", uploadFiles); role uploadFiles(req, res) { console.log(req.body); } app.mind(5000, () => { console.log(`Server started...`); });
Limited contains the bodyParser
object, which is a middleware for populating req.trunk
with the submitted inputs on a form. Calling app.employ(limited.json())
executes the middleware on every request made to our server.
The API is ready with app.post('/upload_files', uploadFiles)
. uploadFiles
is the API controller. Every bit seen above, we are simply logging out req.trunk
, which should be populated by epxress.json()
. We'll examination this out in the case below.
Running body-parser
in Limited
In your last, run node server
to start the server. If washed correctly, you'll see the following in your concluding:
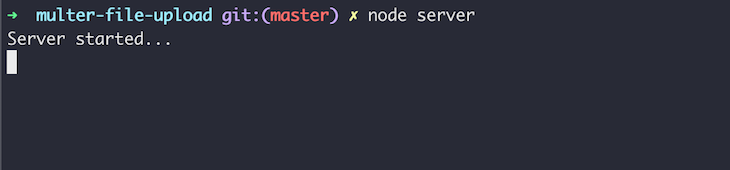
You can now open up your frontend app in your browser. Fill in both inputs in the frontend, the name and files, then click submit. On your backend, yous should meet the following:
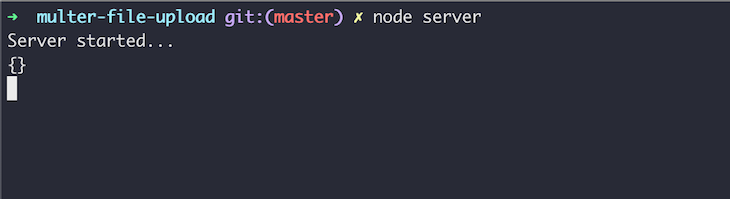
The lawmaking in the image above means that the req.trunk
object is empty, which is to be expected. If you'll call back, trunk-parser
doesn't support multipart
data. Instead, we'll employ Multer to parse the grade.
Install and configure Multer
Install Multer by running the post-obit control in your terminal:
npm i multer
To configure Multer, add together the following to the pinnacle of server.js
:
const multer = require("multer"); const upload = multer({ dest: "uploads/" }); ...
Although Multer has many other configuration options, nosotros're only interested in thedest
property for our project, which specifies the directory where Multer will save the encoded files.
Side by side, we'll apply Multer to intercept incoming requests on our API and parse the inputs to brand them available on the req
object:
app.post("/upload_files", upload.array("files"), uploadFiles); function uploadFiles(req, res) { console.log(req.body); console.log(req.files); res.json({ message: "Successfully uploaded files" }); }
To handle multiple files, utilise upload.array
. For a unmarried file, use upload.unmarried
. Note that the files
argument depends on the name of the input specified in formData
.
Multer volition add the text inputs to req.body
and add the files sent to the req.files
array. To see this at piece of work in the last, enter text and select multiple images on the frontend, so submit and bank check the logged results in your final.
Equally yous can see in the example below, I entered Images
in the text
input and selected a PDF, an SVG, and a JPEG file. Below is a screenshot of the logged upshot:
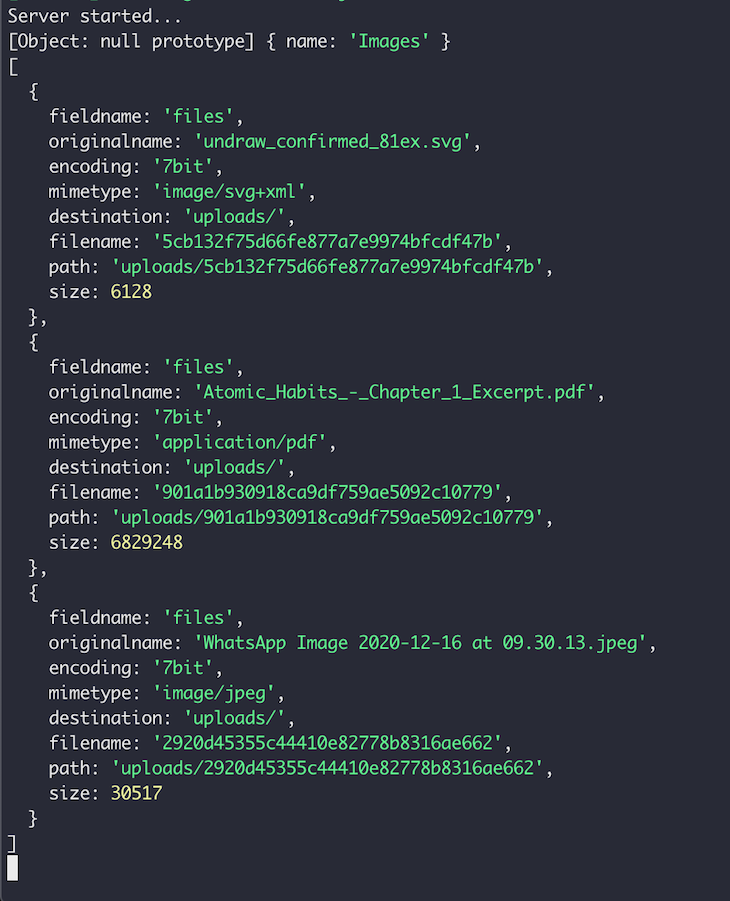
For reference, if you want to upload to a storage service like Cloudinary, you will take have to send the file direct from the uploads binder. The path
holding shows the path to the file.
Conclusion
For text inputs alone, the bodyParser
object used within of Limited is enough to parse those inputs. They make the inputs available as a key value pair in the req.body
object. Multer comes in handy when forms incorporate multipart
data that includes text inputs and files, which the body-parser
library cannot handle.
With Multer, you lot can handle unmarried or multiple files in addition to text inputs sent through a form. Recollect that you should merely use Multer when you're sending files through forms, because Multer cannot handle any form that isn't multipart.
In this article, we've seen a brief of grade submissions, the benefits of trunk parsers on the server and the function that Multer plays in handling course inputs. We also built a small application using Node.js and Multer to see a file upload process.
For the next steps, you can wait at uploading to Cloudinary from your server using the Upload API Reference. I hope you enjoyed this commodity! Happy coding!
200's only
Monitor failed and slow network requests in production
Deploying a Node-based web app or website is the easy part. Making certain your Node case continues to serve resources to your app is where things go tougher. If you're interested in ensuring requests to the backend or tertiary party services are successful, try LogRocket. https://logrocket.com/signup/
LogRocket is like a DVR for spider web and mobile apps, recording literally everything that happens while a user interacts with your app. Instead of guessing why problems happen, you can aggregate and report on problematic network requests to quickly understand the root cause.
LogRocket instruments your app to record baseline operation timings such every bit page load time, time to showtime byte, slow network requests, and also logs Redux, NgRx, and Vuex actions/country. Beginning monitoring for free.
Source: https://blog.logrocket.com/uploading-files-using-multer-and-node-js/
Posted by: raythertualong.blogspot.com
0 Response to "How To Upload Exe As A Web Application"
Post a Comment